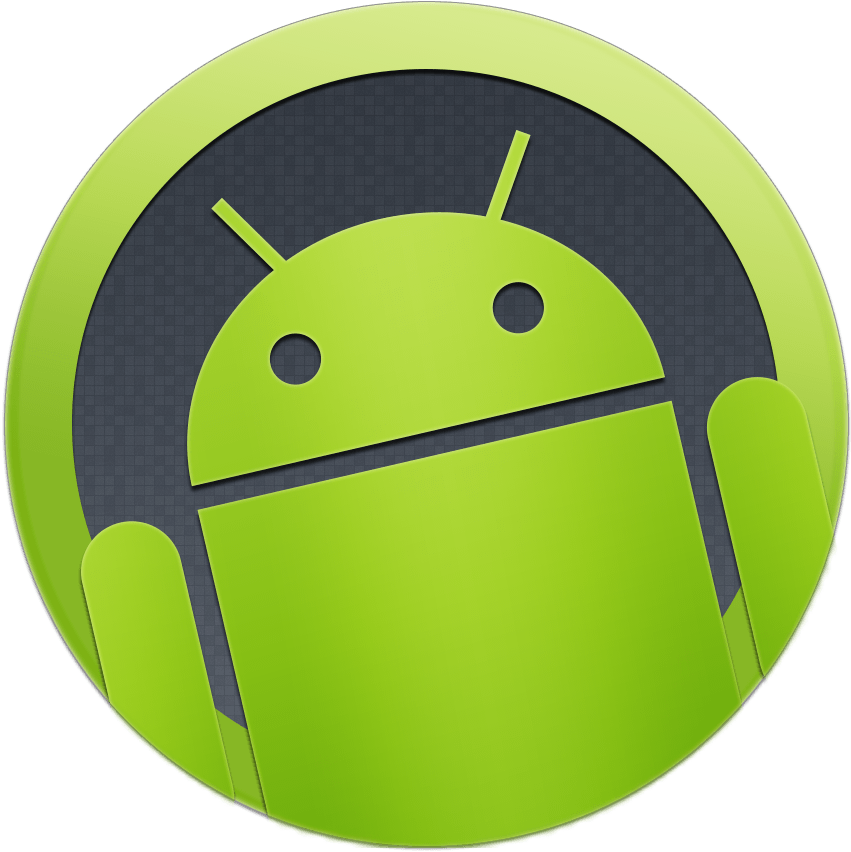
Volley is an HTTP library developed by Google and was first introduced during Google I/O 2013. This library is used to transmit data over the network. It actually makes networking faster and easier for Apps. In this article, we will explain to you how you can download file using Android volley
Volley is available through AOSP(Android Open Source Project) repository. Like most HTTP libraries that are ready to use implementation, you can make a custom request using the volley library.
Volley manages the processing and caching of network requests and it saves developers valuable time from writing the same network call/cache code again and again. It is not suitable for large download or streaming operations since Volley holds all responses in memory during parsing.
Follow the steps below to download file using android volley.
First, we need to do is create a custom request class that extends the Volley request class. To download the file data we can create a custom byte array request. This byte array can be converted into an input stream that will write data to the SDCard.
class InputStreamVolleyRequest extends Request<byte[]> {
private final Response.Listener<byte[]> mListener;
private Map<String, String> mParams;
//create a static map for directly accessing headers
public Map<String, String> responseHeaders ;
public InputStreamVolleyRequest(int method, String mUrl ,Response.Listener<byte[]> listener,
Response.ErrorListener errorListener, HashMap<String, String> params) {
// TODO Auto-generated constructor stub
super(method, mUrl, errorListener);
// this request would never use cache.
setShouldCache(false);
mListener = listener;
mParams=params;
}
@Override
protected Map<String, String> getParams()
throws com.android.volley.AuthFailureError {
return mParams;
};
@Override
protected void deliverResponse(byte[] response) {
mListener.onResponse(response);
}
@Override
protected Response<byte[]> parseNetworkResponse(NetworkResponse response) {
//Initialise local responseHeaders map with response headers received
responseHeaders = response.headers;
//Pass the response data here
return Response.success( response.data, HttpHeaderParser.parseCacheHeaders(response));
}
}
Now send a request through custom class with Request.Method.GET and enter the URL from which you want to download the file from.
String mUrl= <YOUR_URL>;
InputStreamVolleyRequest request = new InputStreamVolleyRequest(Request.Method.GET, mUrl,
new Response.Listener<byte[]>() {
@Override
public void onResponse(byte[] response) {
// TODO handle the response
try {
if (response!=null) {
FileOutputStream outputStream;
String name=<FILE_NAME_WITH_EXTENSION e.g reference.txt>;
outputStream = openFileOutput(name, Context.MODE_PRIVATE);
outputStream.write(response);
outputStream.close();
Toast.makeText(this, "Your Download is Complete.", Toast.LENGTH_LONG).show();
}
} catch (Exception e) {
// TODO Auto-generated catch block
Log.d("KEY_ERROR", "UNABLE TO DOWNLOAD FILE");
e.printStackTrace();
}
}
} ,new Response.ErrorListener() {
@Override
public void onErrorResponse(VolleyError error) {
// TODO handle the error
error.printStackTrace();
}
}, null);
RequestQueue mRequestQueue = Volley.newRequestQueue(getApplicationContext(), new HurlStack());
mRequestQueue.add(request);
Now go to your application folder data/data/<your_application>/<file_name> and there is your file you can also download the file to external storage.
You can access the files in internal storage using
Context.getFilesDir().<file_name>
It returns the file with that name from the internal directory of application and null if there is no file with such name. Dont forget to add extenion of the file with the name.
As you may know, volley does not support large downloads, for large download operations, consider using an alternative like DownloadManager
.